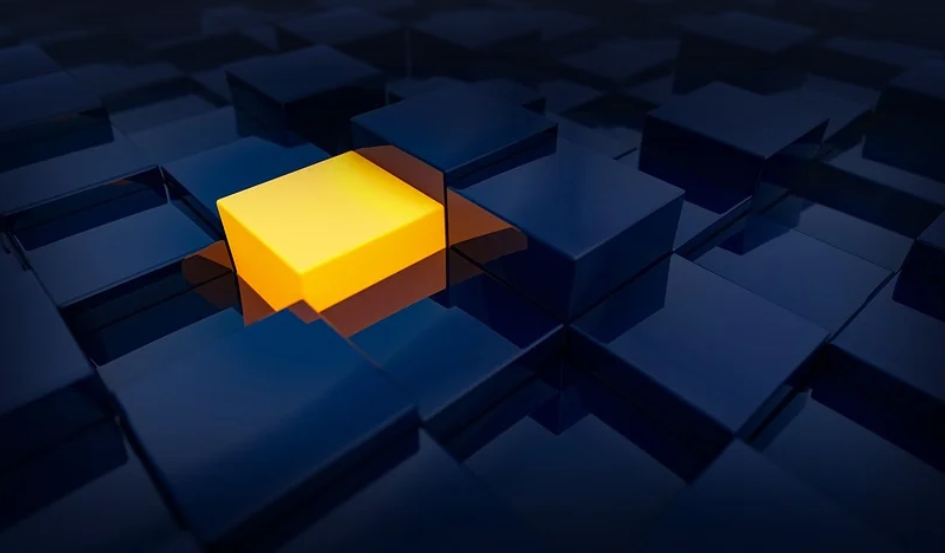
What is Object-Oriented Programming (OOP) and Why Learn It?
Imagine trying to build a house from scratch. You start by assembling individual pieces, like bricks, windows, and doors. Each piece has its own design, specific function, and can be easily moved or swapped out if needed. This is the essence of object-oriented programming (OOP), a powerful approach to building software.
In OOP, we break down complex problems into smaller, more manageable components known as “objects.” These objects are like blueprints for real-world entities – a customer, a product, or even a weather report. Each object has its own data (attributes) and actions (functions) that define its behavior.
Think of it like this: your phone is a complex machine with many components. The “screen” is a component with specific functions – to display images and videos; the “battery” stores energy; and the “microphone” captures sound. OOP allows you to design and manage these individual components, their interactions, and their overall behavior in an organized way.
Why C++ for OOP?
C++ is a powerful and versatile programming language often considered the “gold standard” of object-oriented languages. It’s known for its speed, flexibility, and direct control over hardware and memory.
Let’s examine some key reasons why C++ is a top choice:
- Memory Management: C++ gives you fine-grained control over memory allocation and deallocation, allowing for efficient management of dynamic data structures.
- Flexibility: You can seamlessly blend low-level programming with high-level OOP principles, making it ideal for complex systems.
- Performance: C++ offers unmatched speed and efficiency, crucial in applications where performance is critical like game development or scientific simulations.
- Extensibility: You can write modular code with reusable components that can be expanded upon easily. This promotes rapid development and reduces the chances of creating redundant features.
How does a C++ OOP Structure work?
C++ uses two fundamental principles to create and manage objects: classes and inheritance.
**Classes:** Think of a class as a blueprint for building objects. It defines the attributes (the “state”) and methods (the “behavior”) that your object will have
Imagine a “car” class, where you define its attributes like “color,” “model,” “speed” and functions such as “accelerate,” “brake,” “turn.” Each object you create from this class will have its own specific details based on the blueprint.
**Inheritance:** Inheritance is a powerful mechanism that allows objects to inherit characteristics from other objects. If your car class defines different options, like “sports car” or “pickup truck,” you can create specialized classes (subclasses) with their unique properties.
For example, a “sports car” subclass might inherit the car’s attributes and add its own specific characteristics like “turbocharger” and “aerodynamic design.” This allows for streamlined development, leveraging existing features while adding custom functionality.
Diving into C++ OOP: A Step-by-Step Guide
Let’s dive in with a simple example to illustrate how the above principles work.
We’ll create a basic program that represents a “Car” object, allowing you to modify speed, color, and model.
“`c++ #include This example will help you understand how to create your own classes and objects in C++. You can then go on to explore more advanced concepts like polymorphism, encapsulation, and interface design. Learning object-oriented programming (OOP) is like unlocking a whole new world of software development possibilities. The “PDF” you’re referring to is likely a great resource for further exploration. To make the most out of your learning journey, here are some pointers: Once you master the fundamentals, continue exploring advanced concepts like design patterns and best practices for writing robust, maintainable code. Remember, object-oriented programming is a powerful tool that will empower you to build effective software solutions. Take your time, experiment with different examples, and don’t be afraid to ask questions. As you delve deeper into the world of C++ OOP, you’ll unlock new levels of creativity and efficiency in your development process. Deciphering Object-Oriented Programming with the PDF
Mastering C++ OOP: A Journey Worth Taking